CSCI 1300
Assignment 4: Wandering Eyes
Assigned: Thursday October 23,
2003
Due: Tuesday November 4, 2003
This program will display a pair of eyes on your screen which will
track the cursor as you move the mouse. When the program begins,
a graphics window should open containing two elliptical eyes with round
blue irises inside. If the mouse is in the graphics window, each
iris should follow the mouse; more specifically, each iris should move
to an edge of its ellips so that there is a straight line from the
center of the ellipse to the center of the iris to the mouse pointer.
In addition, when the user clicks the left mouse button inside the
window, the left iris should change from blue to red, or from red back
to blue; when the user clicks the right mouse button inside the window,
the right iris should change from blue to red, or from red back to blue.
In Assignment 3, we told you how to design your program by giving you a
list of functions to write. In this assignment, you must do your
own design. We are deliberately telling you what your program should
accomplish, without telling you precisely how the program should be
constructed. The key is to break the problem into smaller
problems and to design compact, useful functions to solve each of the
smaller problems. One clue to your design is that the program
displays two eyes, but the eyes should behave identically except for
the fact that they are centered in different locations on the
screen. Thus, you should be able to use the same code for both
eyes. If you place this code into a function whose arguments
include the coordinates of the eye, then you can call this function (or
these functions) twice, once per eye.
Another goal of Assignment 4 is for you to learn and use the graphics
library. Using existing libraries is an important part of
programming, because it allows you to leverage code others have
written. You should be able to get enough information about how
the graphics library works from the examples we do in class and from
the documentation available on the web (via the course syllabus page).
When you open a graphics window, it provides a coordinate system for
drawing. The upper left corner is the coordinate (0,0), and the
lower right corner is (XMAX-1, YMAX-1), where XMAX and YMAX are
variables you pick and use as arguments to the initwindow function.
Let's call this coordinate system the pixel
coordinates.
For this assignment, you'll find it useful to use an additional
coordinate system, one in which the center of an eye is the (0,0)
coordinate. Let's call this the Cartesian
coordinates. If (cx, cy) is the center of
the eye in pixel coordinates, then to convert (x,y) from Cartesian
coordinates to pixel coordinates, you use (x+cx, y+cy).
To convert (x,y) from pixel coordinates to Cartesian coordinates, you
use (x-cx, y-cy).
Here is some of the mathematics that should help:
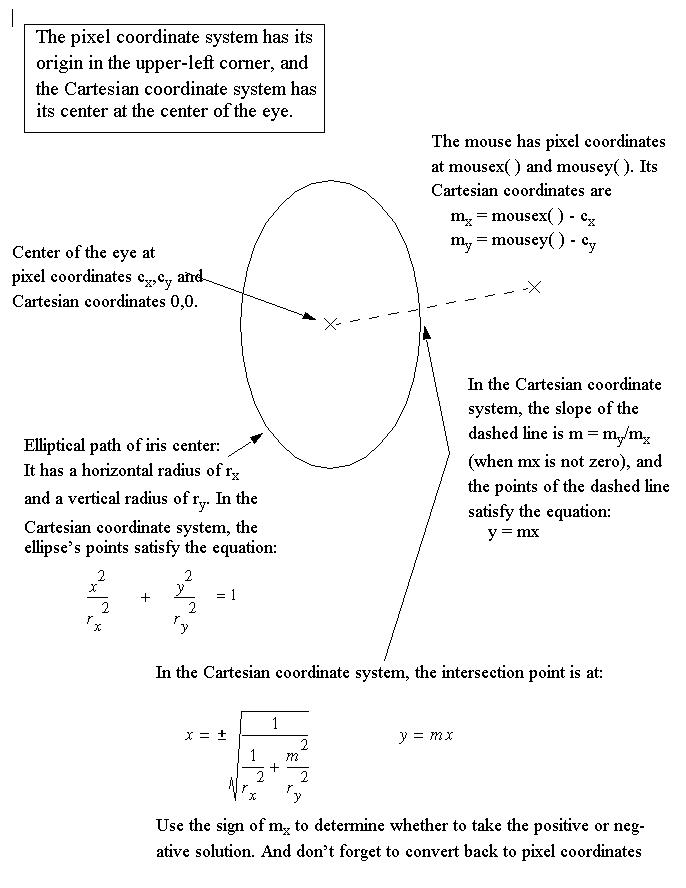
The math above shows you how to pick the coordinates for the center of
the iris. Note, however, that the ellipse in the figure is the
elipse around which the center of the iris should move, not the ellipse
that makes up the eye itself. The ellipse that makes up the eye
is larger. If (ex, ey)
are the radii of the eye itself, and f
is the radius of the iris, then variable rx = ex - f and the variable ry = ey - f.
Getting started
- If you are feeling a bit lost, here are a series of warm-up
exercises you can do that will solve different parts of Assignment
4. Once you've done these relatively simple warm-up exercises,
tackling the main assignment should be straightforward.
(1) Write a program to open
a graphics window, draw two white ellipses (for the eyes). Pick
radii and center locations for the eyes so that they are pleasing to
you. Then add code to draw round blue irises in the center of
each eye. The functions that you will find useful for this
program include: initwindow(), fillellipse(), and
setfillstyle(). Note that drawing a colored circle can use
fillellipse() with the same value for the x and y radii.
(2) Write a program to open a
graphics window, read the mouse location, and draw a white disk (a
filled circle) in that
location. Put this code inside a loop so it continually draws a
disk at the current mouse location. Once you have this
program working, you will have
more confidence in using the mouse functions. The graphics
functions you will find useful for this program include: mousex()
and mousey().
(3) Modify the last program so that as the disk leaves behind no trace
as it moves. To do this, you need to erase the disk at its
previous location. You can erase the last disk by
redrawing it in the color of the background of the window. Note
that you will have to keep track of the current disk center and the last disk center. When you
update the disk, first draw a disk at the last location in the
background color, then draw a white disk in the current location.
(4) Modify the last program by making the program change the color of
the circle if the user left-clicks on the mouse. Graphics
functions you may want to use for testing to see if the mouse button
has been clicked include: ismouseclick() and clearmouseclick().
- Check out the examples we did in class, including the cannon.cxx,
circles2.cxx, and pong1.cxx. I will go over pong1.cxx in
class on Tue Oct 28, but to get started on the assignment, download the
program, and figure out what it does, and compile and run it.
Remember that you will have to follow the directions in the graphics
documentation to include the relevant graphics libraries.
- If you want your TA or Prof. Mozer to review your program design,
we will be happy to go over it with you. You should bring us the
functions you propose to write to decompose the problem, along with
their input and ouputs and a description (in English) of what each
function should do.
- If you are feeling lost, one good way to proceed is to realize
that each variable in the equations above should have a corresponding
variable in your program, and that you will need separate variables for
each eye (e.g., the center of each iris, the center of each eye).
Once you have defined all the variables you need to do the assignment,
it will be easier to think about the input-output behavior of your
functions.
Handing in the assignment
The assignment is due Tuesday November 4, but you can continue to work
on it until 11:00 p.m. November 5 with no penalty. The program
must be submitted via webct.
However, you must have a program you can demo to your TA in recitation
on November 5. Come to recitation with a copy of your program, and you
will have to compile and run it to demonstrate to your TA. If you
have done any bells and whistles, you can show your TA at that
point.
Please be clear: Even though we're giving you a 1 day extension
for handing in the assignment, you must have your assignment working by
the time of your recitation. If it does not work in recitation,
but you do get it working by the time you must submit it to webct,
write your TA a comment at the top of the program explaining what
didn't work in recitation and what works ok in the final version.
As you did in the previous assignments, include your student ID #, your
identikey login name, and your full name in a comment at the top of the
program.