Argus
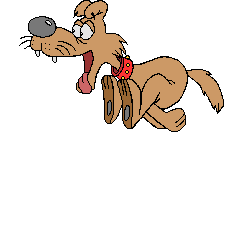
Argus is a dog who lives in the small world shown above.
He is the yellow 3/4 of a circle. The open part of the circle is his mouth, which is always open because he is always happy. In the world
shown here, he is facing east, and he is inside his small house. There
are a few other things in his world: a bone (which is the grey oval in
the top right corner) and a door (which are the double vertical lines
at the west end of Argus's house). Argus can obey five commands:
- turn (which makes Argus turn 90 degrees, clockwise)
- forward (which makes Argus move forward one spot--but don't do this if there's a wall or closed door in front of him!)
- bark (which can only be done if the door is directly in front of Argus, and Argus is not holding his bone; barking changes the door from open-to-shut or from shut-to-open)
- pickup (which makes Argus pickup his bone; this can only be done if Argus is on top of the bone and not holding it already)
- drop (which makes Argus drop his bone; this can only be done if he is holding his bone)
If you try to do anything illegal, then Argus will crash and a big red X appears in his world.
The Argus Environment
I have written a small programming environment to let you control Argus. When you run the environment, you can control Argus directly by pushing buttons for turn, forward, bark, pickup and drop. If you crash the system, then you can press reset to get things going again.
You can also write a program to control Argus, using the Argus Programming Language described below. You can save Argus Programs to a disk file with the save button, or you can load a file from the disk with the load button. To run a program, click the run button. While a program is running, you can make it run faster or slower with the speed bar. You can also pause the execution or stop the execution with two other buttons. If you want to allow recursion in your programs, then the "Allow Recursion" box must be checked on.
To run the Argus Environment on your Windows machine:
- You'll need to make sure that the Java Development Kit is
installed on your machine. The PCs in the Engineering
Center already have this installed, so you can skip
this step there. At home, you can get the JDK from
java.sun.com/products/archive/. On my old (and low memory) home
machine, I have installed the Java 2 SDK
Version 1.2.2_17.
- Open a command window by clicking Start, Run, and then typing cmd
and clicking okay. Normally, this window opens in your own
Documents and Settings Directory. You can then type these commands
to create a new folder for your Argus work, and move into that
folder:
mkdir argus
cd argus
- Download the following file by holding down the left shift key
while you click on this link:
Argus.java.
You should put this file into the argus directory that you
created int he previous step.
- Back to the command window. Type this command to
compile the Argus program:
javac Argus.java
If you are working at home, you might have to add
folder information to the front of the javac command. (On my home
machine, I type c:\jdk1.2.2\bin\javac). You can ignore any warnings
that you get about deprecated APIs.
- From this same directory, execute Argus with the command:
java -classpath . Argus
Notice the space followed by a period followed by another space in the
command. This tells java that the Argus.java program is in the
current folder. From home, you might have to add folder information
to the front of the java command.
The Argus Programming Language
Here is a description of the Argus Programming Language. Keywords and symbols are written in boldface. Programs may also contain comments that start with // and
continue to the end of a line.
- procedure
- An Argus Program is a series of one or more procedures. One of the procedures must be called "main" and this procedure will be executed when the program is run. The format of a procedure is:
procedure name
{
a sequence of statements
}
The "name" may be any non-keyword starting with a letter and followed by a sequence of letters and digits.
- statement
- A statement may be an assert-statement, an if-statement, an if-else-statement, a while-statement, a procedure-call, a block-statement, or one of these five basic statements:
turn;
forward;
bark;
pickup;
drop;
- assert-statement
- An assert-statement has the form:
assert(boolean-expression);
- if-statement
- An if-statement has this form, where the statement may be a block-statement if you like:
if (boolean-expression) statement
- if-else-statement
- An if-else-statement has this form, where each statements may be a block-statement if you like:
if (boolean-expression) statement else statement
- while-statement
- A while-statement has this form, where the statement may be a block-statement if you like:
while (boolean-expression) statement
- procedure-call
- A procedure-call is the name of one of your procedures followed by a semi-colon.
-
block-statement
- A block-statement is an opening curly bracket, followed by a sequence of zero or more statements, followed by a closing curly bracket.
- boolean-expression
- A boolean-expression can be any of the basic tests listed
below. Two expressions can be combined with the operators &&
(and) or || (or). The symbol ! may be put in front of a test to
reverse its meaning.
atBone
atDoor
atX1
atX2
atX3
atX4
atY1
atY2
atY3
hasBone
true
false
facingNorth
facingEast
facingSouth
facingWest
isInside
isClear
Most of these names are self-explanatory, except perhaps
isClear. The isClear predicate is true if Argus can move
forward without running into a wall or door. Some longer examples:
- isInside && hasBone (Argus is inside his house and
he has his bone in his mouth)
- isInside || hasBone (Argus is inside his house or he has
his bone in his mouth)
- !isInside (Argus is not inside his house)
The not operator has the highest precedence; && has second highest
precedence; || has the lowest precedence. For example,
!atX1 || hasBone && isInside
is the same as
(!atX1) || (hasBone && isInside).
Program Ideas
- Assume that Argus starts in the reset position (in the west corner of his
house, facing west, with the door shut).
Write a program that will cause Argus to go to the northwest corner of the
world.
- Rewrite the previous program so that it works regardless of the
starting position. In other words: Regardless of where Argus starts,
the program will take Argus to the northwest corner of the world.
- Assume that Argus starts in the reset position (in the west corner of his
house, facing west, with the bone in the northeast corner and the door shut).
Write a program that will cause Argus to go to his bone and pick it up.
- Rewrite the previous program so that after picking up his bone Argus
returns to his house, drops the bone, and shuts the door.
- Write a program with no assumptions so that Argus and the bone may start
anywhere. The program should cause Argus to find the bone, bring it into
his house, drop the bone, and shut the door.
- Assume that Argus's bone starts outside the house.
Argus himself starts at X=3 and Y=2 facing west, and the door is shut.
Write a program that will cause Argus to go get his bone and move it to the
next spot in the world, moving clockwise. Argus then returns to his
original starting position with the door shut.
- Assume that Argus's bone starts at X=4 and Y=1.
Argus himself starts at X=3 and Y=2 facing west, and the door is shut.
Write a program that will cause Argus to move his bone in a clockwise
direction all the way around the house and back to its original position.
Each time that Argus moves the bone one spot, he must return to his original
position with the door shut before venturing out to move the bone one
more spot.
- Here's a tough one, but one of my favorites: At the start of this
program, Argus can be anywhere in his world, and the world itself
might be larger than the simple 3x4 world that we're used to. Write a
program that will cause Argus to move forward as far as possible, then
turn around and return to his original starting point (but facing in
the opposite direction).
Michael Main
(main@colorado.edu)